Warning
You are reading an old version of this documentation. If you want up-to-date information, please have a look at 2.0 .Sample program
Here we introduce an example of a program to perform a basic pick and place.
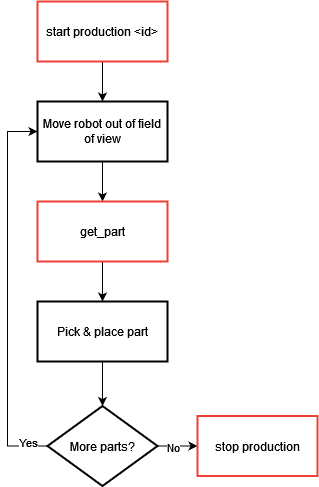
Fig. 6 Production scenario with get_part
It is necessary at this stage to have already performed the camera configuration, as well as the creation of a recipe with its hand-eye calibration.
Basic scenario - get_part
Initialization
1. Prepare the robot
Initialize robot frames and tools.
Initialize the robot gripper.
Move the robot out of the field of view.
2. Initialize the communication
Call the command EYE_CONFIGURE with the correct IP address and port number.
3. Start EYE+ in production
Stop the current state of the EYE+ if needed and start the production with the desired recipe.
Cyclic part of robot program
4. Get the part coordinates
Call the command EYE_GET_PART to get the coordinates of the part to be picked. These coordinates will be stored in the variable EYEPos.
5. Check if no error occurs while requesting the part coordinates
Call the command EYE_CHECK_LAST_ERROR and check if it returns 0. If it does not, an error has occurred (e.g., a timeout)
6. Calculate position
Create the needed positions:
Pick position:
EYEPos
with the Z, RX and RY coordinates,Pick position with Z offset: Define a Z-offset from the pick position to make sure to not hit anything when picking the part, whether it is the Asycube or another part.
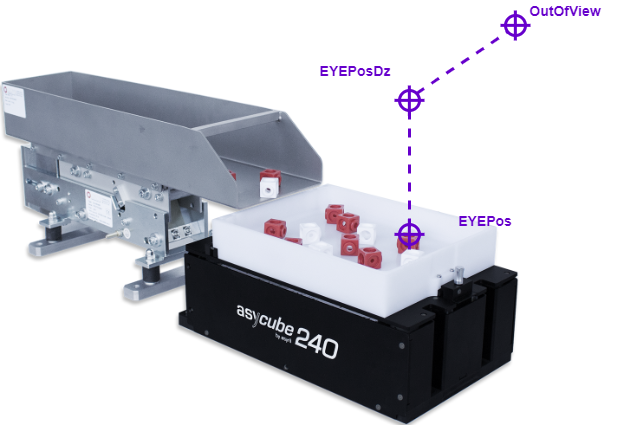
Fig. 7 Path to pick the part
7. Pick
Follow the path from OutOfView
to EYEPosDZ
to EYEPos
and pick the part with your gripper.
8. Place
Follow the path from EYEPos
to EYEPosDZ
to OutOfView
to PlacePos
and place the part with your
gripper.
Example of Denso program
Here is an example of a simple pick and place in PacScript:
#include "densoEyeplusPlugin.pcs"
sub Main
' Take control of the robot arm with default work and tool
takearm keep = 0
' Use the right work and tool for robot pick and place
changework 1
changetool 1
' Move to initial home position
move P, J[0]
' Initialize communication configuration (must be done at least once)
call EYE_CONFIGURE("192.168.0.50", 7171)
if EYE_CHECK_LAST_ERROR() <> 0 then
printmsg("[EYE+] Wrong socket configuration")
exit sub
end if
' Move to initialized position (OutOfView)
move P, P[0]
' Start EYE+ in production
call EYE_START_PRODUCTION(40077)
if EYE_CHECK_LAST_ERROR() <> 0 then
printmsg("[EYE+] cannot start production")
exit sub
end if
'' vvvvvvvv Start pick and place vvvvvvvv ''
for i = 1 to 20
' EYE+ get part
call EYE_GET_PART()
if EYE_CHECK_LAST_ERROR() <> 0 then
' If an error occurred, raised an error and abort
printmsg("[EYE+] Error: " + toString("", eye:EYELastErr))
exit sub
else
' Calculate the pick positions
LetZ EYEPos = // enter z-offset
LetRx EYEPos = // = 180, = 0, ..
LetRy EYEPos = // = 180, = 0, ..
' Pick the part
Approach L, EYEPos, 50
call GripperON()
Move P, EYEPos
call GripperOFF()
' Place the part
Depart L, 50
Approach L, PlacePos, 50
Move P, PlacePos
call GripperON()
Depart L, 50
end if
next
' Move to home position
move P, J[0]
// Stop EYE+
call EYE_STOP("production")
if EYE_CHECK_LAST_ERROR() <> 0 then
printmsg("[EYE+] Cannot stop production")
exit sub
end if
end sub