Sample program
Here we introduce an example of a program to perform a basic pick and place.
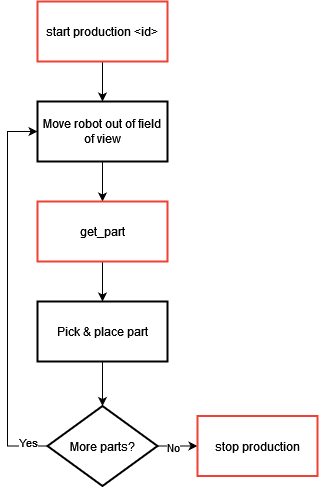
Fig. 10 Production scenario with get_part
It is necessary at this stage to have already performed the camera configuration, as well as the creation of a recipe with its hand-eye calibration.
Basic scenario - get_part
Initialization
1. Prepare the robot
Initialize robot frames and tools.
Initialize the robot gripper.
Move the robot out of the field of view.
2. Initialize the communication
Call the command EYE_CONFIGURE with the correct number of clients.
3. Start EYE+ in production
Stop the current state of the EYE+ if needed and start the production with the desired recipe.
Cyclic part of robot program
4. Get the part coordinates
Call the command EYE_GET_PART to get the coordinates of the part to be picked. These coordinates will be stored in the variable PEYEPos.
5. Check if no error occurs while requesting the part coordinates
Call the command EYE_CHECK_LAST_ERROR and check if it returns 0. If it does not, an error has occurred (e.g. a timeout).
6. Calculate position
Create the needed positions:
Pick position:
PEYEPos
with the Z, A and B coordinates,Pick position with Z offset: Define a Z-offset from the pick position to make sure not to hit anything when picking the part, whether it is the Asycube or another part.
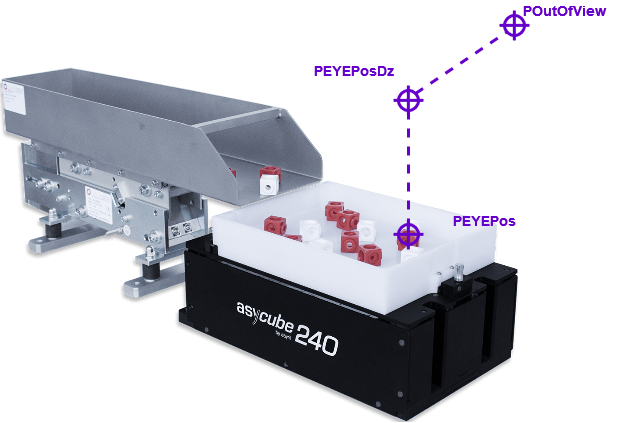
Fig. 11 Path to pick the part
7. Pick
Follow the path from POutOfView
to PEYEPosDZ
to PEYEPos
and pick the part with your gripper.
8. Place
Follow the path from PEYEPos
to PEYEPosDZ
to POutOfView
to PPlacePos
and place the part with your
gripper.
Example of Mitsubishi program
Here is an example of a simple pick and place program:
#Include "EYEPLUSLIB"
' Initialize socket proprieties
EYE_CONFIGURE( 3, 4 )
MLastError = EYE_CHECK_LAST_ERROR()
If MLastError <> 0 Then
' wrong client numbers
EXIT
EndIf
' Initialize components A and B of EYEPos
PEYEPos.A = Rad(180)
PEYEPos.B = 0
PEYEPos.Z = 350
' Move out of field of view
Mov POutOfView
' Start EYE+ in production
EYE_START_PRODUCTION(1)
MLastError = EYE_CHECK_LAST_ERROR()
If MLastError <> 0 Then
' production not started
EXIT
EndIf
' vvvvvvvv Start pick and place vvvvvvvv
For I = 1 To 20 Step 1
' EYE+ get part
EYE_GET_PART()
' If an error occurred, raised an error and abort
MLastError = EYE_CHECK_LAST_ERROR()
If MLastError <> 0 Then
' error while requesting a part
EYE_STOP("production")
'EXIT
Else
' Open the gripper
' ...
' Move above the part
Mov PEYEPos, - 50
' Move on the part
Mvs PEYEPos
' Close the gripper
' ...
' Move above the part
Mvs PEYEPos, - 50
' Move out of field of view
Mov POutOfView
' Place the part
Mov PPlace
' Open the gripper
' ...
' Move out of field of view
Mov POutOfView
EndIf
Next
EYE_STOP("production")
If MLastError <> 0 Then
' error while stopping the production
EXIT
EndIf