Warning
You are reading an old version of this documentation. If you want up-to-date information, please have a look at 2.0 .Sample program
Here we introduce an example of a program to perform a basic pick and place.
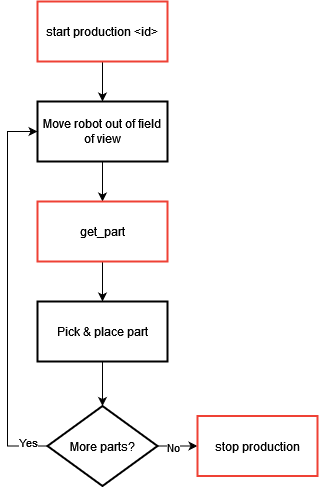
Fig. 16 Production scenario with get_part
It is necessary at this stage to have already performed the camera configuration, as well as the creation of a recipe with its hand-eye calibration.
Basic scenario - get_part
Initialization
1. Prepare the robot
Initialize robot work objects and tools.
Initialize the robot gripper.
Move the robot out of the field of view.
2. Initialize the communication
Call the command EYE_CONFIGURE with the correct communication settings.
3. Start EYE+ in production
Stop the current state of the EYE+ if needed and start the production with the desired recipe.
Cyclic part of robot program
4. Get the part coordinates
Call the command EYE_GET_PART to get the coordinates of the part to be picked. These coordinates will be stored in the variable EYEPos.
5. Check if no error occurs while requesting the part coordinates
Call the command EYE_CHECK_LAST_ERROR() and check if it returns 0. If it does not, an error has occurred (e.g. a timeout).
6. Calculate position
Create the needed positions:
Pick position: Assign the required elements EYEPos.trans.z, EYEPos.rot of the position EYEPos,
Pick position with Z offset: Define a Z-offset from the pick position to make sure not to hit anything when picking the part, whether it is the Asycube or another part.
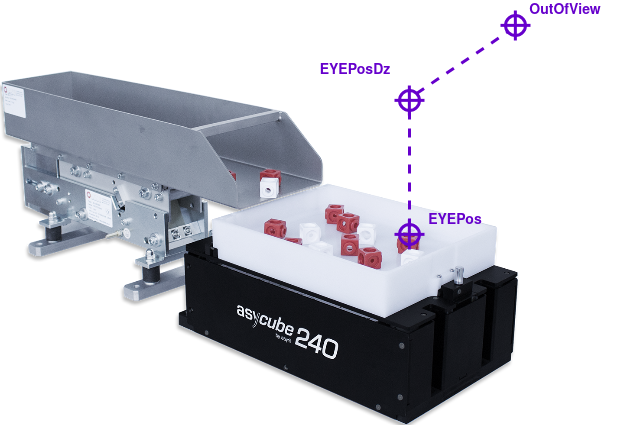
Fig. 17 Path to pick the part
7. Pick
Follow the path from OutOfView
to EYEPosDZ
to EYEPos
and pick the part with your gripper.
8. Place
Follow the path from EYEPos
to EYEPosDZ
to OutOfView
to PlacePos
and place the part with your
gripper.
Example of ABB program
Here is an example of a simple pick and place program:
MODULE SampleProgram
! Define all required positions
VAR robtarget EYEPosDz;
VAR robtarget PosOutofView;
VAR robtarget PlacePart;
PERS tooldata my_gripper; ! you must define your gripper
VAR num DZ := 20;
PROC main()
PosOutofView.trans := [0, 200, 200];
PosOutofView.rot := OrientZYX(0, 0, 180);
MoveL PosOutofView, v200, z0, my_gripper;
! Configure the communication with right settings / Initialize position EYEPOS
EYE_CONFIGURE "192.168.0.50", 7171;
IF EYE_CHECK_LAST_ERROR() <> 0 THEN ! Check that no error on last command
EXIT;
ENDIF
! Define static orientation and orientation to pick the parts
EYEPos.rot := OrientZYX(0,0,180);
EYEPos.trans.z := 100;
! Start EYE+ in production state with right recipe identifier
EYE_START_PRODUCTION 12345;
IF EYE_CHECK_LAST_ERROR() <> 0 THEN ! Check that no error on last command
EXIT;
ENDIF
! Start a loop for 20 picks and places
FOR i FROM 1 TO 20 DO
! Ask for a part coordinates
EYE_GET_PART;
IF EYE_CHECK_LAST_ERROR() <> 0 THEN ! Check that no error on last command
ErrWrite \I, "Error while trying to get one part coordinate", "";
EXIT;
ELSE
! Define an intermediate position EYEPosDz to reach the target with DZ offset
EYEPosDz := EYEPos;
EYEPosDz.trans.z := EYEPosDz.trans.z + DZ;
! Move to intermediate position
MoveJ EYEPosDz, v100, z0, my_gripper;
WaitRob \InPos;
! Move to position
MoveL EYEPos, v50, z0, my_gripper;
WaitRob \InPos;
! Pick the part with your gripper
! Move to intermediate position
MoveJ EYEPosDz, v100, z0, my_gripper;
WaitRob \InPos;
ENDIF
! Place the part
MoveJ PlacePart, v100, z0, my_gripper;
WaitRob \InPos;
ENDFOR
! Stop EYE+ production state and stop the communication
EYE_STOP "production";
IF EYE_CHECK_LAST_ERROR() <> 0 THEN ! Check that no error on last command
EXIT;
ENDIF
ENDPROC
ENDMODULE