Sample program
Here we introduce an example of a program to perform a basic pick and place.
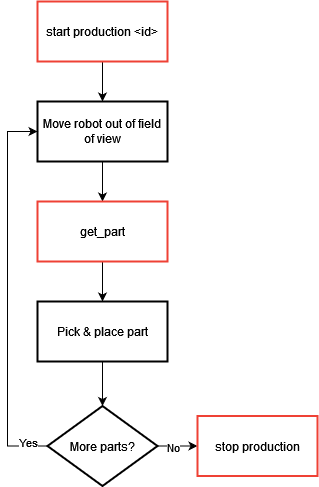
Fig. 12 Production scenario with get_part
It is necessary at this stage to have already performed the camera configuration, as well as the creation of a recipe with its hand-eye calibration.
Basic scenario - get_part
Initialization
1. Prepare the robot
Initialize robot frames and tools.
Initialize the robot gripper.
Move the robot out of the field of view.
Note
Refer to section Robot initialization program example for more details.
2. Initialize the communication
Call the commands:
E_RESET in your start.pgx program,
E_CONFIGURE with the correct IP address and port number.
3. Start EYE+ in production
Stop the current state of the EYE+ if needed and start the production with the desired recipe.
Cyclic part of robot program
4. Get the part coordinates
Call the command E_GET_PART to get the coordinates of the part to be picked. These coordinates will be stored in the variable EYEPos_Rx / EYEPos_Rs.
5. Check if no error occurs while requesting the part coordinates
Call the command E_CHECK_ERR and check if it returns 0. If it does not, an error has occurred (e.g., a timeout)
6. Calculate position
Create the needed positions:
Pick position:
eye:EYEPos_Rx
with the Z, RX and RY coordinates,Pick position with Z offset: Define a Z-offset from the pick position to make sure to not hit anything when picking the part, whether it is the Asycube or another part.
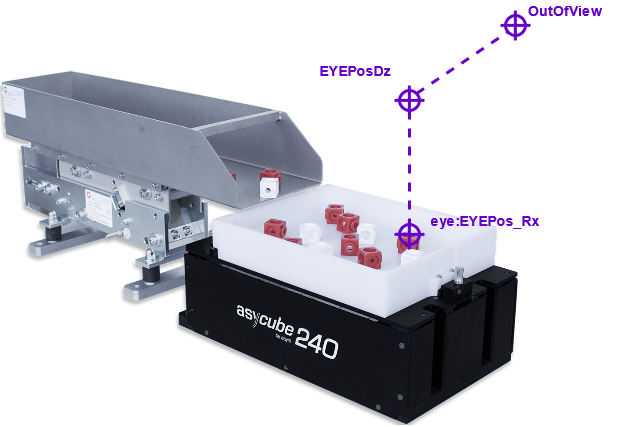
Fig. 13 Path to pick the part
7. Pick
Follow the path from OutOfView
to EYEPosDZ
to eye:EYEPos_Rx
and pick the part with your gripper.
8. Place
Follow the path from eye:EYEPos_Rx
to EYEPosDZ
to OutOfView
to PlacePos
and place the part with your
gripper.
Example of Stäubli program
Here is an example of a simple pick and place in SRS:
// Reset plugin global variables (must be done at least once)
call eye:E_RESET()
// Initialize socket properties (must be done at least once)
call eye:E_CONFIGURE("192.168.0.50", 7171)
call eye:E_CHECK_ERR()
if eye:EYELastErr != 0
logMsg("[EYE+] Wrong socket configuration")
return
endIf
// Initialize robot properties (frame, tool, positions)
call init()
// Move to initialized position
movej(pOutOfView, tCalibration, mDesc)
waitEndMove()
// Start EYE+ in production
call eye:E_START_PROD(32439)
call eye:E_CHECK_ERR()
if eye:EYELastErr != 0
logMsg("[EYE+] cannot start production")
return
endIf
// vvvvvvvv Start pick and place vvvvvvvv //
for i = 1 to 20
// EYE+ get part
call eye:E_GET_PART()
call eye:E_CHECK_ERR()
if eye:EYELastErr != 0
// If an error occurred, raised an error and abort
logMsg("[EYE+] Error: " + toString("", eye:EYELastErr))
return
else
// Calculate the pick positions
eye:EYEPos_Rx.trsf.z = // enter z-offset
eye:EYEPos_Rx.trsf.rx = // = 180, = 0, ..
eye:EYEPos_Rx.trsf.rz = -eye:EYEPos_Rx.trsf.rz
// Pick the part
movel(appro(eye:EYEPos_Rx, {0,0,nuOffset,0,0,0}), tCalibration, mDesc)
movel(eye:EYEPos_Rx, tCalibration, mDesc)
waitEndMove()
// Venturi on
dioOutputValve1[0] = true
// Place the part
movel(appro(eye:EYEPos_Rx, {0,0,nuOffset,0,0,0}), tCalibration, mDesc)
movej(pOutOfView, tCalibration, mDesc)
movel(pPlacePos, tCalibration, mDesc)
waitEndMove()
// Venturi off
dioOutputValve1[0] = false
// Move out of field of view
movel(pOutOfView, tCalibration, mDesc)
endIf
endFor
// Stop EYE+
call eye:E_STOP("production")
call eye:E_CHECK_ERR()
if eye:EYELastErr != 0
logMsg("[EYE+] cannot stop production")
return
endIf