Sample program
Here we introduce an example of a program to perform a basic pick and place.
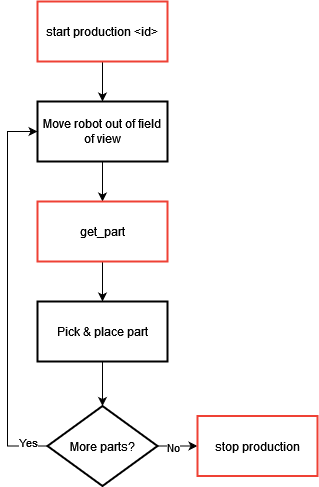
Fig. 9 Production scenario with get_part
It is necessary at this stage to have already performed the camera configuration, as well as the creation of a recipe with its hand-eye calibration.
Basic scenario - get_part
Initialization
1. Prepare the robot
Initialize robot frames and tools.
Initialize the robot gripper.
Move the robot out of the field of view.
2. Initialize the communication
Call the commands EYE_CONFIGURE with the correct IP address, port number and starting point.
3. Start EYE+ in production
Stop the current state of the EYE+ if needed and start the production with the desired recipe.
Cyclic part of robot program
4. Get the part coordinates
Call the command EYE_GET_PART to get the coordinates of the part to be picked.
These coordinates will be stored in the point P[StartPoint]
.
5. Check if no error occurs while requesting the part coordinates
Call the command EYE_CHECK_LAST_ERROR and check if it returns 0. If it does not, an error has occurred (e.g., a timeout).
6. Calculate position
Create the needed positions:
Pick position: Copy
P[StartingPoint]
toP043
with the Z, RX and RY coordinates,Pick position with Z offset:
P041
with a Z-offset from the pick position to make sure not to hit anything when picking the part, whether it is the Asycube or another part.
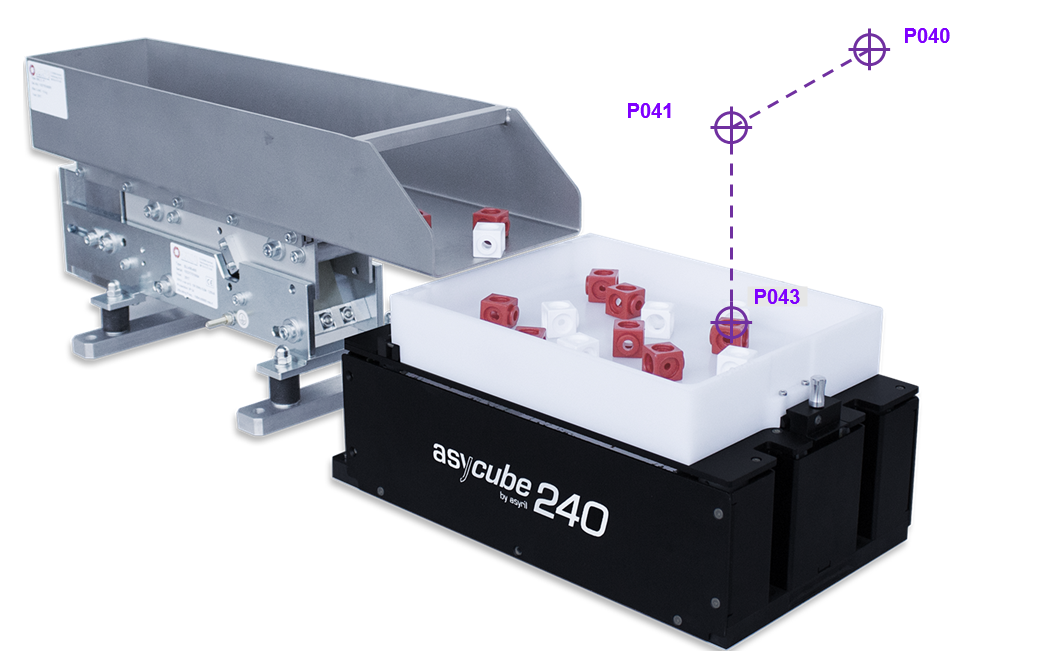
Fig. 10 Path to pick the part
7. Pick
Follow the path from P040
to P041
to P043
and pick the part with your gripper.
8. Place
Follow the path from P043
to P041
to P042
and place the part with your gripper.
Example of Yaskawa program
Here is an example of a simple pick and place in MotoSim with starting point to 300 :
NOP
'-----------------------
' Example of integration
'-----------------------
'
' Prepare your
' - User coordinates
' - Tool
' - Gripper
'
' EYE+ conf: 192.168.0.50:7171
' Reserved memory area: 300-600
CALL JOB:EYE_CONFIGURE ARGF "192.168.0.50" ARGF 7171 ARGF 300
'
' Check if no error while configuring the communication
CALL JOB:EYE_CHECK_LAST_ERROR
GETS LI010 $RV
IFTHEN LI010<>0
JUMP *stop
ENDIF
'
' Start EYE+ in production
CALL JOB:EYE_START_PRODUCTION ARGF 65447
'
' Check if no error while starting the production
CALL JOB:EYE_CHECK_LAST_ERROR
GETS LI010 $RV
IFTHEN LI010<>0
JUMP *stop
ENDIF
'
' Move out of field of view
MOVL P040 V= 10.0
'
'
CALL JOB:EYE_CHECK_LAST_ERROR
GETS LI010 $RV
IFTHEN LI010=0
' Start pick and place
'--- LOOP 20 ---
SET I298 0
WHILE I298<20
' EYE+ get_part
CALL JOB:EYE_GET_PART
'
' If no error: pick and place
CALL JOB:EYE_CHECK_LAST_ERROR
GETS LI010 $RV
IFTHEN LI010<>0
JUMP *stop
ENDIF
'
' Calculate positions
SET P043 P300
' Set Z-value
SETE P043 (3) -16500
' Set RX value
SETE P043 (4) 1790000
' Set RY value
SETE P043 (5) 0
'
' Move to approache point
SET P041 P043
SETE P041 (3) 30000
MOVL P041 V= 10.0
'
' Move on the part
MOVL P043 V= 10.0
'
' Pick the part - Gripper ON
DOUT OT#(1) ON
TIMER T=0.200
'
' Move to approache point
MOVL P041 V= 10.0
'
' Place the part - Gripper OFF
MOVL P042 V= 10.0
DOUT OT#(1) OFF
' Move out of field of view
MOVL P040 V= 10.0
INC I298
ENDWHILE
ENDIF
'
' Stop EYE+
*stop
CALL JOB:EYE_STOP ARGF "production"
'
' Check if no error while stopping the production
CALL JOB:EYE_CHECK_LAST_ERROR
GETS LI010 $RV
IFTHEN LI010<>0
'error
ENDIF
'
END