Warning
You are reading an old version of this documentation. If you want up-to-date information, please have a look at 4.2 .Plugin functions
You can start programming with the EYE+ plugin on the IPendant touch through TPE programs.
Important
The camera configuration and hand-eye calibration must be done from the EYE+ Studio interface before executing any commands. If you do not know how to proceed, please refer to the Camera configuration wizard and Hand-eye calibration wizard sections.
To call a plugin command from a TPE program, you must use the CALL
command and select the program through
[INST]/CALL/CALL program/COLLECT/KAREL Progs
.
Important
The EYE_COMM1 and EYE_COMM2 commands are private programs. These programs are used internally to manage the communication with your EYE+. Please do not call these two programs.
The following 7 commands are basic programs allowing to create a simple and easy to integrate pick and place.
EYE_CONFIGURE
EYE_STOP
EYE_START_PRODUCTION
EYE_GET_PART
EYE_PREPARE_PART
EYE_RAW_COMMAND
EYE_CHECK_LAST_ERROR
EYE_CONFIGURE(client1 = 1, client2 = 2)
Parameters
client1 - is the client number of the first client you configured in step Plugin configuration. The parameter must be an integer between 1 and 8. If no parameter is given as input, client1 will be set to 1.
client2 - is the client number of the second client you configured in step Plugin configuration. The parameter must be an integer between 1 and 8. This parameter must not be the same as the first one. If no parameter is given as input, client2 will be set to 2.
Description
This command must be called at the beginning of your program to specify the clients used by the communication.
Note
If you have not configured the clients correctly in step Plugin configuration, you will get the
following error 602 Client configuration error
and the communication between your robot and your EYE+ will not
start.
Usage example
1: EYE_CONFIGURE(1, 2)
3: EYE_CHECK_LAST_ERROR
4: IF (R[21:Asyril-EYELastEr] = 602) THEN
5: MESSAGE('Not well configured')
6: ENDIF
EYE_START_PRODUCTION(recipe_id)
Parameters
recipe_id - the recipe’s unique identifier. The parameter must be an integer between 1 and 65535.
Description
This command must be called to start EYE+ in production state using the right recipe.
Usage example
1: EYE_CONFIGURE(1, 2)
2: EYE_START_PRODUCTION(12345)
3: EYE_CHECK_LAST_ERROR
4: IF (R[21:Asyril-EYELastEr] = 0) THEN
5: MESSAGE('EYE+ is in production')
6: ENDIF
7: EYE_STOP('production')
EYE_STOP(state)
Parameters
state - either
production
,recipe_edition
,camera_configuration
orhandeye_calibration
. The parameter must be a string.
Description
This command is used to stop an EYE+ state and stop the communication on both clients. You must always end your
program with an EYE_STOP('production')
.
Warning
If you miss the call at the end of your program, you will need to do a fnct/ABORT (ALL)
with the
IPendant touch to stop the communication when starting another program.
Usage example
1: EYE_CONFIGURE(1, 2)
2: EYE_STOP('recipe_edition')
3: EYE_STOP('camera_configuration')
4: EYE_STOP('handeye_calibration')
5: EYE_STOP('production')
6:
7: EYE_START_PRODUCTION(12345)
8: ...
9:
10: EYE_STOP('production')
11: EYE_CHECK_LAST_ERROR
12: IF (R[21:Asyril-EYELastEr] = 0) THEN
13: MESSAGE('EYE+ is in ready state')
14: ENDIF
EYE_GET_PART(pos_reg = 20)
Parameters
pos_reg - (optional) is the position register number to which the coordinates of the found part will be written. If no parameter is specified as input, the default position register used is
PR[20:Asyril-EYEPos]
. The parameter must be an integer.
Returns
The returned part is stored in the input position register.
Only the X, Y and R components are overwritten in the specified position register. You have to add the other components (Z, W, P) yourself according to your robot setup. These missing components can be assigned by hand or by using the assignment operator in your program.
Warning
If you do not assign the last components, the position will not be reachable by your robot.
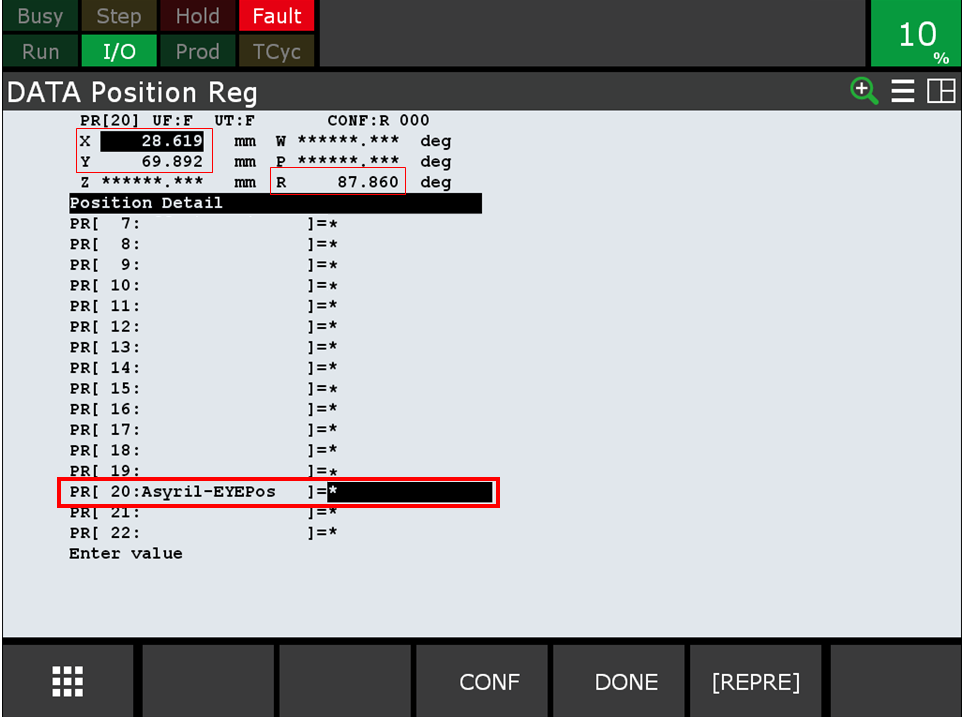
Fig. 211 Position register if you do not fill in the components Z, W, P
Description
This command is used to request one part from EYE+. This is a blocking command, meaning it will keep going until it gets a response from EYE+.
Usage example
1: EYE_CONFIGURE(1, 2)
2: EYE_START_PRODUCTION(12345)
3:
4: //Assign the other components of the part position to be reachable
5: PR[20:Asyril-EYEPos, 3] = 15
6: PR[20:Asyril-EYEPos, 4] = (-180)
7: PR[20:Asyril-EYEpos, 5] = 0
8:
9: EYE_GET_PART
10: EYE_CHECK_LAST_ERROR
11: IF (R[21:Asyril-EYELastEr] = 0) THEN
12: MESSAGE('EYE+ found a part')
13: ENDIF
14: EYE_STOP('production')
EYE_PREPARE_PART
Description
This command is used to request one part from EYE+. This command is not a blocking command. The part coordinates can be retrieved later with the EYE_GET_PART command.
Usage example
1: EYE_CONFIGURE(1, 2)
2: EYE_START_PRODUCTION(12345)
3:
4: //Assign the other components of the part position to be reachable
5: PR[20:Asyril-EYEPos, 3] = 15
6: PR[20:Asyril-EYEPos, 4] = (-180)
7: PR[20:Asyril-EYEpos, 5] = 0
8:
9: EYE_PREPARE_PART
10:
11: ...
12:
13: EYE_GET_PART
14: EYE_CHECK_LAST_ERROR
15: IF (R[21:Asyril-EYELastEr] = 0) THEN
16: MESSAGE('EYE+ found a part')
17: ENDIF
18: EYE_STOP('production')
EYE_RAW_COMMAND(cmd, str_reg = 20, client = 1)
Parameters
cmd - is the raw command to send to EYE+. This parameter must be a string.
str_reg - (optional) is the register number to which the response to the raw command will be written. If no parameter is specified as input, the default string register used is the
SR[20:Asyril-EYERawRes]
register. The parameter must be an integer.client - (optional) is the client number that will be used to send the command. The parameter must be an integer whose value is equal to 1 or 2. If no parameter is specified as input, the default client is the 1.
Note
Client 1 is the first client you entered in the EYE_CONFIGURE command and client 2 is the second client you entered.
Warning
If you want to specify the client number, you must also specify the str_reg. If you don’t, you may have unexpected issues.
Returns
The raw response of the command is stored in the string register str_reg.
Description
This function is used to send raw commands to EYE+. Refer to chapter Commands to know what kind of commands can be sent.
Warning
If you send the command EYE_RAW_COMMAND('set_parameter part_quantity 2')
, the
EYE_GET_PART command will provide only the first part coordinates in
the position register. However, if you use a EYE_RAW_COMMAND('get_part')
command, the string response will
contain all the part coordinates.
Usage example
1: EYE_CONFIGURE(1, 2)
2: EYE_RAW_COMMAND('start production 12345`)
3: IF (SR[20:Asyril-EYERawRes] = '200') THEN
4: MESSAGE('EYE+ is in production')
5: ENDIF
6: EYE_STOP('production')
EYE_CHECK_LAST_ERROR(int_reg = 21)
Parameters
int_reg - (optional) is the register number used to store the last detected error. The parameter must be an integer. If not specified, the register used is the
R[21:Asyril-EYELastEr]
register.Warning
Make sure the int_reg parameter is not a reserved register. Refer to Table 37 for further information.
Returns
The last error detected is stored in the register number int_reg.
Description
This function is used to check if an error has occurred.
If no error has occurred, the output is equal to 0.
If an EYE+ error has occurred, the output is equal to one of the error codes listed in Error codes (error type
4xx
or5xx
).If a plugin error has occurred, the output is one of the following errors displayed in Table 35 (error type
6xx
).
Error code |
Signification |
---|---|
601 |
Communication error |
602 |
Client configuration error |
603 |
Connection failed |
604 |
Timeout occurred |
605 |
Wrong client numbers |
606 |
Wrong input parameters |
699 |
Generic error |
Once the error is returned as output from the function, the error is cleared internally (value set to 0).
Usage example
1: EYE_CONFIGURE(1, 2)
2: EYE_GET_PART
3: EYE_CHECK_LAST_ERROR
4: IF (R[21:Asyril-EYELastEr] = 403) THEN
5: MESSAGE('Production subsystem has not been started')
6: ENDIF
7: EYE_CHECK_LAST_ERROR
8: IF (R[21:Asyril-EYELastEr] = 0) THEN
9: MESSAGE('Now the error is cleared')
10: ENDIF
11: EYE_STOP('production')